It is continuous process to either move web pages or content in Web Application development over the years. Search Engine Optimization and page hit count, getting more traffic for the website is the most important things in day today, moving pages or ceasing pages might not find the new page hits in search engines.
For example: Imagine that you have categories of products in your E-Commerce web application. When the old categories are replaced or updated by new categories, each year a page in your site might be replaced or updated accordingly. If any external sites (or even internal pages) have links to the category of these web pages in your web application, when link is clicked for the next time then it would have thrown error.
You would typically use Reponse.Redirect to suppress the error. But this is a manipulation, not actual work. This will work for the visitor but it internally 302 redirect.
Response.Redirect
In previous versions of ASP.NET we are using Response.Redirect that redirects to HTTP 302 found status response in turn redirects the page URL temporarily to the specified page URL. This is extra overhead to the server, performance hit here.
Problems
1. There is a round trip here.
2. 302 redirect is temporary redirect for the page.
3. No Search Engine Optimization (SEO) benefits due to this approach
4. You are neither informing search engine to updated internal indexes nor pass old page rank to new page.
Solution: Issuing permanent HTTP 301 redirect will solve the purpose, directly available in ASP.NET 4.0
Importance of HTTP Response Code 301:
This happens to move some WebPages or entire website from one domain to another or change the URL of some of the pages inside the website most of the time. This is quite difficult for a webmaster who deletes the pages from the old server, maps them to the new domain. In this case webmaster looses SEO that is related to previous links. In order to prevent SEO loss we need to make use of 301 Redirects.
HTTP Response Code 301 instructs the browser that the particular page has been moved permanently from the old location to the new location. The benefit of using 301 Redirects is that the search engine, crawlers store SEO information related to the old link based on 301 redirects. It also informs the search engines that the page location has permanently moved and it needs to index the page from the new location in future. This is really useful for search engines like Bing or Google that will carry over page rank to the new page if this status code is seen.
Response.RedirectPermanent
It’s the method of Page.HTTPReposne class that permanently redirects a requested URL to specified URL and issue HTTP 301response code Moved permanently responses. It uses the URL given by the location header. Unnecessary round trip for the browser is eliminated using this feature. This is used in migrating websites from old domain to new domain especially. As inbound traffic is important for significant usage of the web application this option is very much useful in live web applications as the requested URL is permanently redirected to some location using this mechanism. Since the redirection is HTTP 301 response status, search engine knows the new link that is redirected and results in the redirect.
Syntax :
RedirectPermanent("/newlocation/oldcontent.aspx");
A Practical Approach
Install Visual Studio 2010 Beta Download and Installation Steps
Open VS2010 and Go to File -> New-> Project ->Visual C Sharp->Web-> Empty ASP.NET Web Application.
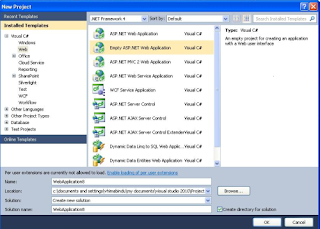
Right click on the Project Properties and click on Add-> New Item ->NewWebForm Name it as OldForm.aspx . Here are the screen shots
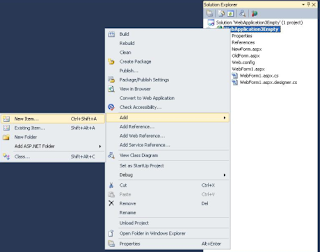
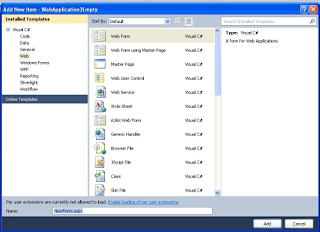
Drag and Drop the Label control and place it in the OldForm.aspx
Change the text in the label as “This is the Old Page”.
Here is the code how it looks
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="OldForm.aspx.cs" Inherits="WebApplication3Empty.OldForm" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>OldForm</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text="Label">This is the Old
Page</asp:Label>
</div>
</form>
</body>
</html>
Again Right click on the Project Properties and click on Add-> New Item ->NewWebForm Name it as NewForm.aspx .Here is the code in the NewForm.
Change the title and Place the text of your choice or as mentioned below in the NewForm.aspx.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="NewForm.aspx.cs" Inherits="WebApplication3Empty.NewForm" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>New Form</title>
</head>
<body>
<form id="form1" runat="server">
<div>
This is New Form to be redirected
</div>
</form>
</body>
</html>
Now in the Webform1.aspx that is added by the project, drag and drop a link button and specify postbackurl property to oldform.aspx
Now, Redirect permanently to NewForm.aspx in the OldForm.aspx Page_Load event.
Here is the code that it looks like .
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication3Empty
{
public partial class OldForm : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
//Redirects to Permanent specified url from the requested url
Response.RedirectPermanent ("~/NewForm.aspx");
}
}
}
That’s it; now click F5 to run the application.
Output: When Webform1.aspx is clicked though it’s redirected to OldPage.aspx, but it permanently 301 redirected to NewPage.aspx from OldPage.aspx.
If you install FireFox HHTP Header plug in https://addons.mozilla.org/en-US/firefox/addon/3829, you can actually trace this by going to Tools -> Live Headers
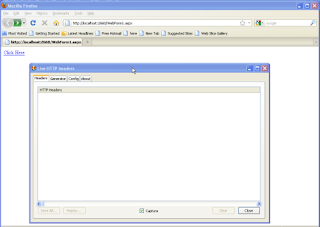
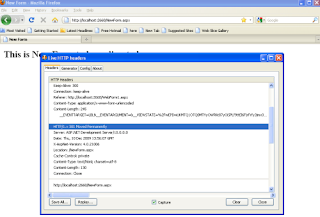
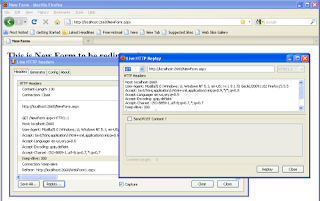
Here is the explanation of how it works .
When WebForm1.aspx link button is clicked, it’s actually redirected to oldpage.aspx, from there page will issue HTTP 301 permanently response code. This initiation again requests the browser that the OldPage.aspx has been moved permanently to NewPage.aspx. Accordingly search engines is notified that it needs to index the page from NewPage.aspx here after.
If you have Fiddler you can debug the application and inspect HTTP headers and HTTP301 redirects clearly.
Here is the screen shot of the out put and how it behaves in the back ground.
Conclusion:
Detailed explanation and practical implementation of RedirectPermanent usage with examples is explained. This is helpful for creating search engine friendly, permanent 301 redirects when developing ASP.NET websites .The feature is simple but its usage is much important for ASP.NET web developers. If you have pages in search engines that are moved to new location, then Response.RedirectPermanent really useful for updating the search engine with the new URL.
5 comments:
Awesome explanation Hima. It's me who raised the doubt in the session. Thanks Hima for your excellent job.
Good Explaination. Its help others alot.
Nice fill someone in on and this mail helped me alot in my college assignement. Gratefulness you seeking your information.
Hai,
Exelent Hima and tks for u r explanation,Keep on postings
good one ... really useful...
Post a Comment